Guides
Distributing builds to Mac App Store
While most artifacts can be enabled by a simple toggle in ToDesktop's settings, building for Mac App Store (MAS) requires more steps.
This guide explains how to build Mac App Store apps for development and production.
Apple Developer Walkthrough
Create an Apple Developer account. If eligible, consider enrolling in Apple's Small Business Program to reduce your App Store commission fees.
Once done, navigate to the main Apple Developer interface.
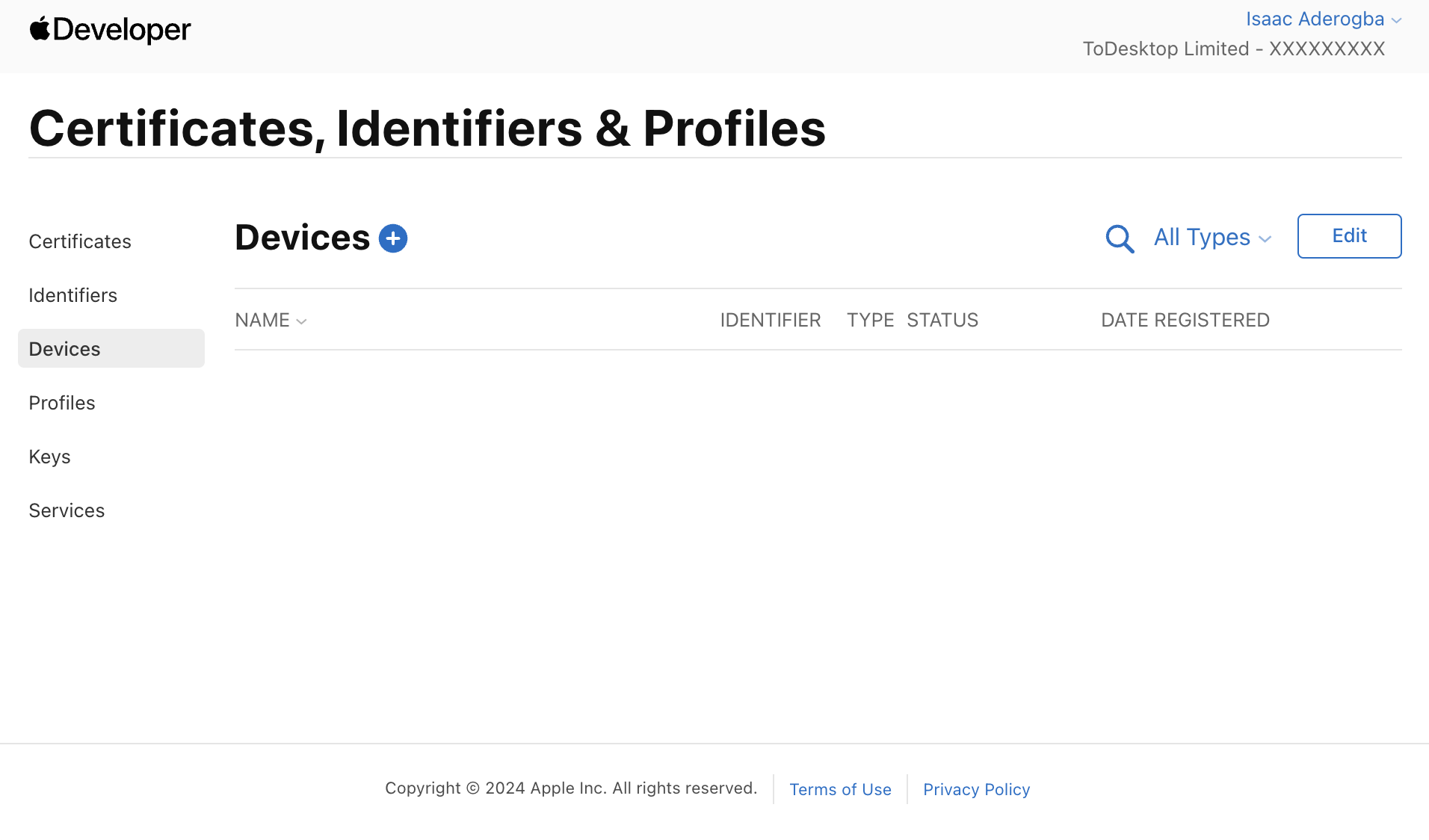
Next, navigate to Devices and add your device to your Developer Account List:
- Click + to add a device and fill in the details.
- To get the Device ID of your Mac, open the System Information app and copy the value next to the Provisioning UDID.
Navigate to Certificates and create the following Mac certificates:
- Apple Development: used to sign apps for development and testing on machines that have been registered on the Apple Developer website.
- Apple Distribution: used to sign apps submitted to the Mac App Store.
- Mac Installer Distribution: used to sign the Mac Installer Package for submission to Mac App Store Connect.
Next, create provisioning profiles to verify your identity as a developer when distributing your app. An App ID is needed for this, so we'll create that first:
On the Identifiers page, register a new App ID:
- Platform: MacOS
- Bundle ID: Explicit. Apple recommends using a reverse-domain name style string (i.e., com.domainname.appname). This does not need to correspond with an actual website.
- When done click continue, review it, then click register.
The App ID is the same appId
that you will provide in your todesktop.json
in a later step:
Next, add the app to App Store Connect:
- Click on My Apps > + (to create a new app) > select New MacOS App > Select the appId you registered previously.
- Fill out the remaining sections.
You can now generate your two provisioning profiles. Navigate to Profiles and do the following:
Generate the AppleDevelopment Provisioning Profile
- Click the + sign to add a new profile.
- Select "Mac App Store".
- Choose the appropriate distribution certificate.
- Give it a recognizable name like "AppleDevelopment", then download it to your computer.
- Double click the file to install it, then place it in the project's build directory. The file name would be AppleDevelopment.provisionprofile. This corresponds to the provisioningProfile key in the package.json file. Whatever you name it, during the build process it gets renamed to embedded.provisionprofile.
Generate the MacAppStore Provisioning Profile
- Click the + sign to add a new profile, select Mac App Store connect, and then select the relevant distribution certificate.
- Select your Mac App Distribution certificate, then click continue.
- Give it a recognizable name like "MacAppStore", then download it to your computer.
- Unlike development profiles, distribution profiles cannot be installed locally. These profiles are used during the Mac App Store submission process. If you try to install them locally, you'll see the following error:
This also means that apps built for distribution cannot be run locally. If you want to test your apps before submission to the Mac App Store, they must be built for development.
ToDesktop Walkthrough
With your certificates in hand, navigate to ToDesktop and go to Settings > Build and Deploy. Enable the Mac App Store artifact.
Next, click on the Certificates tab and upload your Apple certificates:
- Mac App Store Development Certificate accepts your downloaded Apple Development certificate.
- Mac App Store Distribution Certificate accepts your downloaded Apple Distribution certificate.
- Mac App Store Installer Certificate accepts your Mac Installer Distribution certificate.
ToDesktop also supports Developer ID Certificates for building other Mac distribution formats, such as DMGs or ZIPs.
Code Walkthrough
Finally, update your project's todesktop.json
file with these fields (this example assumes you're building for development):
id
: Your ToDesktop application ID, retrievable in Settings > General.appId
: The App ID that you created on the Apple Developer site.appBuilderLibVersion
: The app-builder version to use when building your app. Specify at least version25.0.0
in your configuration. This version includes important updates for supporting multiple certificates, which are not currently available in the stable release.buildVersion
: The specified build version.icon
: The specified icon.schemaVersion
: The specifiedtodesktop.json
schema version.mac.extendInfo
: Extra entries to be populated in your built app'sInfo.plist
. It's necessary to specifyElectronTeamID
(this is your Apple Developer Team ID).mas.type
: Whether to build Mac App Store for"development"
or"distribution"
.mas.entitlements
: Path to the entitlements file (more on this later).mas.entitlementsInherit
: Path to the child entitlements file (more on this later).mas.provisionprofile
: Path to a provisioning profile you downloaded from the Apple Developer site.
We'll examine the entitlements
and provisionprofile
keys in detail, as they require special attention.
Entitlements
Your application's entitlements declare what permissions and APIs that your app needs to access.
Mac App Store applications must be sandboxed. This requires using a limited set of entitlements from the full list available.
A sample entitlements file, entitlements.mas.plist
, has the following contents:
A sample child entitlements file, entitlementsInherit.mas.plist
, has the following contents:
In most cases, you shouldn't need to adjust the entitlementsInherit.mas.plist
file. If you need to add additional permissions for your app, these should be included in the entitlements.mas.plist
file.
Both files should be present in your repository so that they are used by ToDesktop when building your app.
Provisioning Profile
Use the development or distribution provisioning profile you downloaded earlier.
For development builds, use your development provisioning profile. This allows you to run the application on your local computer. Only the Device IDs that are associated with the provisioning profile will be able to run the app locally.
When uploading your app to the Mac App Store, use your distribution provisioning profile.
Distribution apps cannot be run directly on your local Mac. They are intended only for submission to Apple.
Conclusion
You should now have all the information you need to build apps for Mac App Store. If you run into issues or have any questions, please drop us an email at [email protected].