Introduction
Building an application
Using ToDesktop to manage your Electron app allows you to build, test, and release your app with confidence. To get started, navigate to ToDesktop, click the create new app button, and select the todesktop platform option.
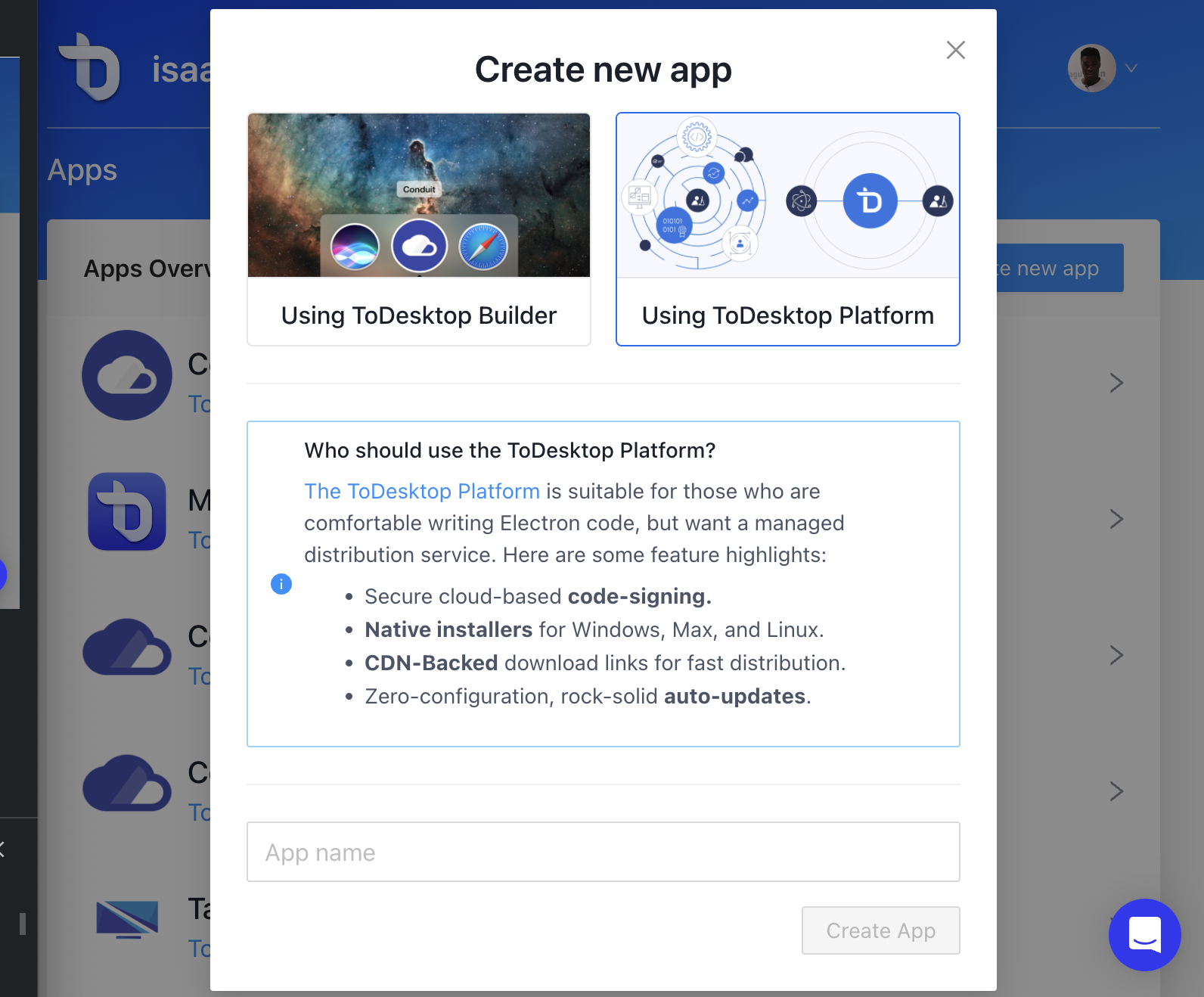
The todesktop builder option is our no-code solution if you'd rather avoid writing Electron code and re-use your existing web application.
Generating an app
If you don't have an existing Electron app, you can use our create-desktop-app NPM package to create a basic application that comes pre-installed with @todesktop/cli
and @todesktop/runtime
:
You can then build your desktop app with the following command:
This will execute the @todesktop/cli
build command and prompt you to log in and enter your registered email address and access token. You can find your access token by clicking your profile dropdown in the web interface and selecting the manage access token option.
Using an existing app
If you have an existing application, you can instead install @todesktop/cli
as a global dependency. The @todesktop/cli
package will take care of authentication and programmatically interacting with the ToDesktop platform.
Create a todesktop.json
file in the root of your Electron project, using the following configuration and changing the properties as needed:
You may optionally want to include the appFiles
property in your todesktop.json. This allows you to decide which files get packed into your app using an array of glob patterns. For example, the following will match all files in the src
directory (any level of nesting) that have the .js
extension. The package.json
, package-lock.json
and yarn.lock
files are always included if they exist.
Next, install the @todesktop/runtime
package as a project dependency. This will take care of application auto-updating, crash reporting, and more:
In your main (background process) script, import the package and call the init
function. The function should be called as early as possible:
You can then proceed to build your desktop app with the following command:
This will first prompt you to log in and enter your registered email address and access token. You can find your access token by clicking your profile dropdown in the web interface and selecting the manage access token option.
As your application is building, progress updates will be streamed to your project terminal (as a response to invoking the build
command) and in the build view on the ToDesktop web interface.
Visit Part 2 of this guide to learn how to sign your build to make it eligible for distribution.