Menus
How to Create Dynamic Tray Menus
Learn how to create dynamic tray menus for your ToDesktop Builder application
Introduction
Adding dynamic menus to your desktop app is simple through the use of the ToDesktop API. In this short walkthrough, we’ll re-create the following menu:
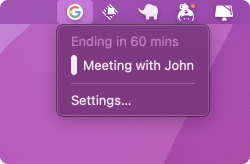
Before continuing, this guide assumes that you’re familiar with extending ToDesktop Builder applications with custom code. For a walkthrough on how to extend an existing application, please visit How to Build a TodoMVC Desktop App with ToDesktop Builder.
Code Walkthrough
We’ll walk step-by-step through creating a buildDynamicMenu
function that does the following:
- Creates the tray.
- Creates the menu.
- Attaches the menu to the tray at a set interval.
Dynamic menu functionality was added in version 1.0.0-alpha43
of @todesktop/client-core
, so please make sure that you are on the latest version.
You can skip to the full implementation at the bottom of the page if you want to see the final code.
To start, we’ll sketch out the body of the function and get our imports out of the way:
Next, we’ll create an electron NativeImage
, passing a base64 data image as input. Importantly, interacting with electron from a web javascript environment involves managing electron references. trayIconRef
and trayRef
are two examples, but you can think of them as pointers to the underlying Electron objects that live in the node.js environment.
In this example, we used a data image that we created ahead of time. For your own projects, you can easily create a data image from an image element or URL source using vanilla JavaScript.
We’ll follow a similar process for creating the icon for our menu item. We’ll also resize it and set it as a Template Image so that it works in light or dark mode.
Directly after, we’ll create a utility function that allows us to set a tray menu. We’re opting to create a function because we’ll be using this in a setInterval
invocation later.
Inside the function's body, we calculate the remaining time for a fictitious meeting. We then create a menuRef
and use that alongside the trayRef
to set the tray’s context menu.
Finally, we’ll execute setTrayMenu
and then re-execute it every 60 seconds.
You could also re-trigger setTrayMenu
based on events that have occurred in your app - there’s no need for an explicit interval. Another important detail is that you must replace the entire menu, as opposed to updating specific menu items. This is because Electron’s rendering logic doesn’t support mutating menus in-place.
That’s it! You now have an example function for creating the following dynamic menu.
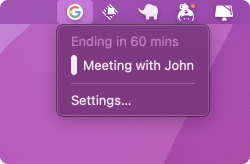